buildfire.components.listView
The listView
component provides a consistent tool to display lists of items featuring a search bar, custom header content, item image shapes, actions, item content, and more.
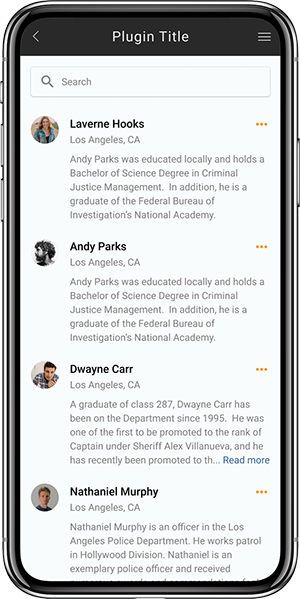
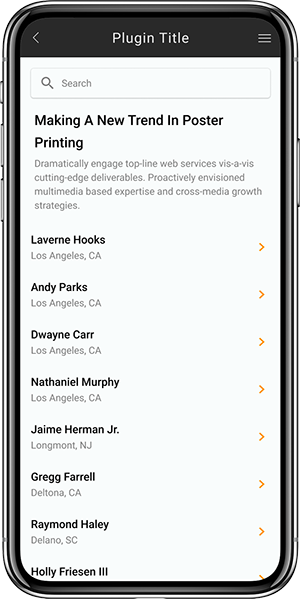
Requirements
Widget
<head>
<!-- CSS -->
<link rel="stylesheet" href="../../../styles/components/listView@2.0/listView.css" />
<!-- JS -->
<script src="../../../scripts/buildfire.min.js"></script>
<script src="../../../scripts/buildfire/components/listView@2.0/listView.js"></script>
</head>
In your widget body create html container element for list view items.
<body>
<div id="listViewContainer"></div>
</body>
Methods
const listView = new buildfire.components.listView(selector, options);
ListView class constructor method which initializes the list view component.
const listView = new buildfire.components.listView('#listViewContainer');
listView()
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
selector | string | yes | CSS selector of element that will contain component | |
options | object | no | options object |
options
Name | Type | Required | Description | Default |
---|---|---|---|---|
settings | object | no | settings object | |
translations | object | no | translations object |
settings
Name | Type | Required | Description | Default |
---|---|---|---|---|
showSearchBar | boolean | no | Hides or shows the search bar | true |
itemImage | string | no | Specifies the shape of the item image circle, square, avatar, none | none |
headerContent | string | no | Custom HTML elements | null |
paginationEnabled | boolean | no | If pagination is enabled onDataRequest provides the page and the pageSize | false |
paginationOptions | object | no | paginationOptions object | |
contentMapping | object | no | contentMapping object | |
customListAction | object | no | customListAction object | |
enableReadMore | boolean | no | Specifies whether the description text should be truncated or not | true |
maxHeight | number | no | Specifies the maximum height of the component | null |
paginationOptions
Name | Type | Required | Description | Default |
---|---|---|---|---|
page | number | no | Specifies the starting page for the data | 0 |
pageSize | number | no | Specifies the page size for the data | 10 |
contentMapping
Name | Type | Required | Description | Default |
---|---|---|---|---|
idKey | string | yes | Specifies the id field of the item | id |
imageKey | string | no | Specifies the image field for the item | imageUrl |
titleKey | string | no | Specifies the title field for the item | title |
subtitleKey | string | no | Specifies the subtitle field for the item | subtitle |
descriptionKey | string | no | Specifies the description field for the item | description |
customListAction
Using customListAction disables passing actions to the list using the onItemRender handler.
Name | Type | Required | Description | Default |
---|---|---|---|---|
html | html | yes | HTML Element to be appended |
translations
Name | Type | Required | Description | Default |
---|---|---|---|---|
readMore | string | no | Specifies the text for the read more button | Read More |
readLess | string | no | Specifies the text for the read less button | Read Less |
emptyStateMessage | string | no | Specifies the empty state message below the empty state image | No Items Yet |
searchInputPlaceholder | string | no | Specifies the text placeholder in the search bar | Search |
append()
listView.append(items, appendToTop)
Appends an item or many items to the list view
const listView = new buildfire.components.listView('#listViewContainer', options);
listView.append([
{
id: '1',
title: 'buildfire',
imageUrl: 'https://via.placeholder.com/150',
subtitle: 'The Most Powerful App Maker For iOS & Android',
description: 'Buildfire’s powerful and easy to use mobile app builder...'
}
]);
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
items | [object] | object | yes | Array of items, or single item to be appended to list view. |
appendToTop | boolean | no | false | Specifies whether items should be applied to the top or the bottom of the list |
update()
listView.update(id, data)
Updates an item and re-renders it in the list
const listView = new buildfire.components.listView('#listViewContainer', options);
listView.update(1, {
id: 1,
imageUrl: 'https://via.placeholder.com/150',
title: 'John Doe',
subtitle: 'CEO',
description: 'Owner of the company'
});
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
id | string | yes | Object id | |
data | object | yes | Object to be updated |
remove()
listView.remove(id)
Removes an item from the list view
const listView = new buildfire.components.listView('#listViewContainer');
listView.remove(1);
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
id | string | yes | Object id |
clear()
listView.clear()
Clears the list view
const listView = new buildfire.components.listView('#listViewContainer', options);
listView.clear();
refresh()
listView.refresh()
Refreshes the component to apply changes and re-render items
const listView = new buildfire.components.listView('#listViewContainer', options);
listView.options.settings.showSearchBar = false;// hides the search bar
listView.refresh();
reset()
listView.reset()
Resets the component to its initial state
const listView = new buildfire.components.listView('#listViewContainer', options);
listView.reset();
Handlers
The following event handlers can be assigned:
onDataRequest(event, callback)
Triggered when:
- The component loads for the first time
- The user scrolls to the bottom and requests the next page
- Calling
refresh()
orreset()
- The user is using the search bar
If you don't want to use this handler you can populate the list using the append method.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
event | object | yes | holds search value and pagination options if pagination is enabled | |
callback | number | no | provides callback to retrieve items back to component for render |
Event
Name | Type | Required | Description | Default |
---|---|---|---|---|
searchValue | string | yes | holds search value from the search bar | null |
page | number | no | If pagination is enabled provides the needed page for the pending fetch | 0 |
pageSize | number | no | If pagination is enabled provides the specified pageSize | 10 |
Callback
Name | Type | Required | Description | Default |
---|---|---|---|---|
items | array | yes | array of items that need to be rendered inside the component |
onItemRender(event)
Triggered every time before an item is rendered, provides a return statement to specify actions for the item and its custom content.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
event | object | yes | event object |
Event
Name | Type | Required | Description | Default |
---|---|---|---|---|
item | string | yes | item that component is currently rendering |
Example
Provides return statement to retrieve actions and custom content for a specific item.
listView.onItemRender = (event) => {
let actions = [
{ actionId: 'remove', text: 'Remove item' },
{ actionId: 'update', text: 'Update item' },
];
if(event.item.age > 36) actions.push({ actionId: 'call', text: 'Call' });
let customContent = 'Some custom content';
return { actions, customContent };
}
Name | Type | Required | Description | Default |
---|---|---|---|---|
actions | array | no | array of action objects | |
customContent | string | no | custom content for the item that will appear below the description |
onItemRendered(event)
Triggered every time after an item is rendered, you can use this handler to execute javascript for the custom content.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
event | object | yes | event object |
Event
Name | Type | Required | Description | Default |
---|---|---|---|---|
item | string | yes | item that component is currently rendering | |
html | html | yes | HTML container for the rendered item |
Example
You can use this handler to execute any functionality needed after the item has been rendered.
listView.onItemRendered = (event) => {
if (event.item.data.age > 33)
buildfire.components.ratingSystem.injectRatings();
}
onRenderEnd(event)
Triggered every time after the component has done rendering batch of items.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
event | object | yes | event object |
Event
Name | Type | Required | Description | Default |
---|---|---|---|---|
items | array | yes | Contains array of rendered items | |
containers | html | yes | Contains array of html containers for rendered items |
Example
You can use this handler to execute any functionality needed after the item has been rendered.
listView.onRenderEnd = (event) => {
//do your logic here
}
onItemClick(event)
Triggered when an item is clicked. Passes the item in the event parameter.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
event | object | yes | event object |
Event
Name | Type | Required | Description | Default |
---|---|---|---|---|
item | object | yes | Item clicked | |
target | string | yes | Specifies what part of the item has been clicked title, subtitle, action |
Using customListAction will pass action as a target parameter.
Example
listView.onItemClick = event => {
console.log('onItemClick', event);
}
onItemActionClick(event)
Triggered when an item's action is clicked. Passes the item and action in the event parameter.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
event | object | yes | event object |
Event
Name | Type | Required | Description | Default |
---|---|---|---|---|
item | object | yes | item interacted with | |
action | object | yes | action clicked |
onSearchInput(searchValue)
Triggered when the users types in something in the search bar.
This handler will not be invoked if onDataRequest is being used
Event
Name | Type | Required | Description | Default |
---|---|---|---|---|
searchValue | string | yes | Contains the search value. |
Example
listView.onSearchInput = (searchValue) => {
console.log('onSearchInput', searchValue);
}
Examples
Using ListView with pagination enabled
Example 1
Using the component with pagination enabled, the component will take care of which page is next to be fetched.
Code
let listView = new buildfire.components.listView("#listViewContainer", {
settings: {
itemImage: 'circle',
paginationEnabled: true,//we enable pagination
paginationOptions: {
page: 0,
pageSize: 5//we set our custom page size
},
contentMapping: {
titleKey: 'data.title',
subtitleKey: 'data.subtitle',
imageKey: 'data.imageUrl',
descriptionKey: 'data.description',
}
}
});
listView.onDataRequest = (event, callback) => {
let searchOptions = {
limit: event.pageSize,
skip: event.page * event.pageSize,
sort: { title: 1 }
}
event.searchValue ? searchOptions.filter = { "$json.title": { $regex: event.searchValue, $options: 'i' } } : null;
getData(searchOptions, (result) => {
callback(result);
});
}
listView.onItemRender = event => {
let actions = [
{ actionId: 'call', text: 'Call' },
{ actionId: 'email', text: 'Email' }
];
if (event.item.age > 36)
actions.push({ actionId: 'remove', title: 'Remove' });
let customContent = "Some custom content...";
return { actions, customContent };
}
listView.onItemActionClick = (event) => {
if (event.action.actionId == 'remove')
listView.remove(options.item.id);
}
Example 2
Using with pagination disabled
Using the component with pagination disabled will not pass page and pageSize in the options parameter of the onDataRequest handler.
Code
let listView = new buildfire.components.listView("#listViewContainer", {
settings: {
itemImage: 'circle',
contentMapping: {
titleKey: 'data.title',
subtitleKey: 'data.subtitle',
imageKey: 'data.imageUrl',
descriptionKey: 'data.description',
}
}
});
listView.onDataRequest = (event, callback) => {
getData(event.searchValue, (result) => {
callback(result);
});
}
listView.onItemClick = event => {
event.item.data.title = 'You clicked me';
event.item.data.description = 'Nothing much to see here';
event.item.data.imageUrl = 'https://via.placeholder.com/150';
event.item.data.age = 33;
listView.update(event.item.id, event.item);
}
Example 3
Using without onDataRequest
Using the component without the onDataRequest handler you will need to populate the component using the append method.
Code
let listView = new buildfire.components.listView("#listViewContainer", {
settings: {
itemImage: 'circle',
contentMapping: {
titleKey: 'data.title',
subtitleKey: 'data.subtitle',
imageKey: 'data.imageUrl',
descriptionKey: 'data.description',
}
}
});
listView.append({
id: "someId",
data: {
title: "John Smith",
subtitle: "Developer",
description: "Javascript developer"
}
});
listView.onSearchInput = (event) => {
getData(event.searchValue, (result) => {
listView.clear();
listView.append(result);
});
}
Example 4
Using with customListAction
In this example, we are using the component with the customListAction feature which disables passing actions in the onItemRender handler.
Code
let listView = new buildfire.components.listView("#listViewContainer", {
settings: {
itemImage: 'square',
contentMapping: {
titleKey: 'data.title',
subtitleKey: 'data.subtitle',
imageKey: 'data.imageUrl',
descriptionKey: 'data.description',
},
customListAction: {
html: `<i class="material-icons">chevron_right</i>`,
}
}
});
listView.onDataRequest = (event, callback) => {
getData(event.searchValue, (result) => {
callback(result);
});
}
listView.onItemClick = event => {
if(event.target === 'action') {
//do something
}
}