buildfire.components.reactions
Reactions
The Reactions component is a versatile tool that can be integrated into features to add reaction functionality to any item, such as posts, reviews, articles, media, and more. The component provides a customizable interface that allows users to interact with available reactions easily while managing all the backend and frontend complexities.
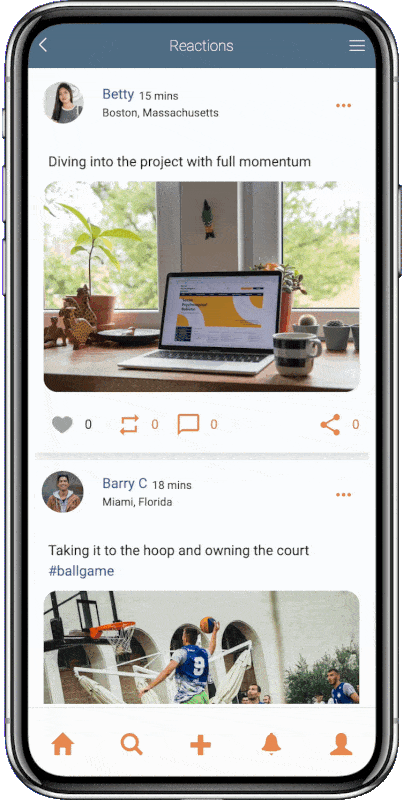
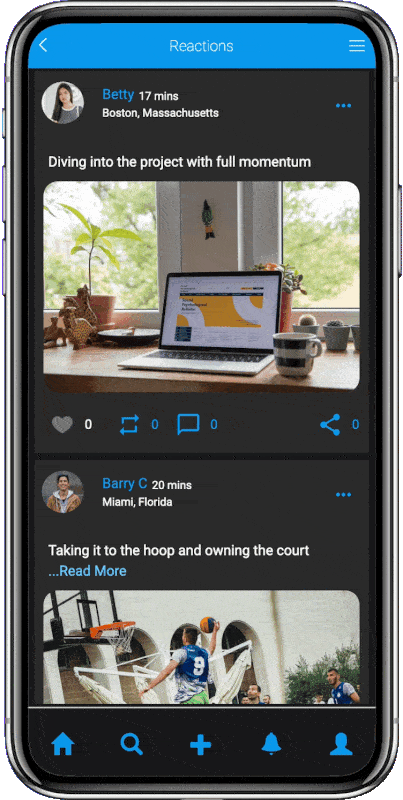
Requirements
Widget
<head>
<!-- CSS -->
<link rel="stylesheet" href="../../../styles/components/reactions/reactions.css" />
<!-- JS -->
<script src="../../../scripts/buildfire.min.js"></script>
<script src="../../../scripts/buildfire/components/reactions/reactions.js"></script>
<!-- If you want to show user reactions details, you should include the drawer component-->
<script src="../../../scripts/buildfire/components/drawer/drawer.js"></script>
</head>
This component requires the Reactions feature to be installed in the app to function properly
It can be found on the Control Panel in the Marketplace
Usage
The Reactions component takes care of fetching and displaying the reactions block into the specified HTML elements, toggling reactions, and showing reaction details.
Reaction
Class constructor method which initializes the reaction component.
reactions()
const reactions = new buildfire.components.reactions(selector<String>, options<Object>)
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
selector | string | yes | A string containing selector to match. This string must be a valid CSS selector string; if it isn't, a SyntaxError exception is thrown. | |
options | object | yes | Options object |
options
Name | Type | Required | Description | Default |
---|---|---|---|---|
itemId | string | yes | Unique ID for the Item we are going to apply the reaction to. | |
itemType | string | no | A string representing the item type. This is useful in cases where different reactions are applied to different item types. | |
groupName | string | no | A string representing the group name of the selected group of reactions defined in the Reactions feature | If nothing is provided, the first available group in the Reactions feature will be used. If a not available group name is provided, it will throw an Error |
showCount | boolean | no | If true , show Total reactions count | true |
showUsersReactions | boolean | no | If true , show Users reactions when pressing on reactions count. Requires showCount to be true | true |
If the user is not logged in, they will be redirected to the login screen once they interact with the reaction component
Methods
injectReactions()
buildfire.components.reactions.injectReactions(selector)
This static method is helpful if you are going to initialize the reactions component in HTML tags instead of creating a new javascript instance of the component as described above. Once called, any HTML element having the component HTML attributes will be rendered.
Make sure to call the injectReactions()
function again each time a new reaction is dynamically added to the DOM
buildfire.components.reactions.injectReactions(selector<String>);
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
selector | string | no | A string containing selector to match. This string must be a valid CSS selector string; otherwise, a SyntaxError exception is thrown. | 'body' |
Handlers
onUpdate()
An event handler function will be called each time a reaction occurs. It will have an event
parameter that is automatically passed.
function reactions.onUpdate(event<Object>)
event
Name | Type | Description |
---|---|---|
status | string | Could be one of the following: add , delete , update . add when performing a reaction, delete when we remove an active reaction, and update when we toggle an old reaction type with a new one |
reactionType | string | The reaction type of the reaction that was executed and fired the event |
itemId | string | Unique ID for the Item where the reaction took place |
userId | string | Unique ID for the logged-in user that made the reaction |
groupName | string | The Reaction group name that the reaction belongs to |
itemType | string | A string representing the item type. This is useful in cases where different reactions are applied to different item types |
reactionId | string | Unique ID for the logged-in user that made the reaction |
onError()
An event handler function that will be called each time a reaction error occurs. It will have an event
parameter that is automatically passed.
function reactions.onError(event<Object>)
event
Name | Type | Description |
---|---|---|
itemType | string | A string representing the item type. This is useful in cases where different reactions are applied to different item types |
itemId | string | Unique ID for the Item where the reaction error took place |
errorCode | string | Error code, reflecting the error type and details |
message | string | Error description |
event.errorCode
Code | Description |
---|---|
1001 | Missing required data |
1002 | Invalid selector provided |
1003 | Provided group name does not exist |
5001 | Server failed to fetch data |
5002 | Server failed to update data |
5003 | Server failed to delete data |
HTML Attributes
The Reaction component could be initialized using HTML attributes. Any DOM Element having those attributes will get the reactions injected into it after calling the injectReactions()
method.
HTML
<div id="reactionsContainer">
<div
bf-reactions-item-id="005589dfaeaab1"
bf-reactions-on-update="onReaction" bf-reactions-on-error="onReactionError" bf-reactions-show-count="true" bf-reactions-show-users-reactions="true">
</div>
</div>
For any dynamically added DOM Element or group of elements having the reactions attributes, we need to call injectReactions()
again for the reactions to be properly injected.
HTML Attributes
Name | Type | Required | Description |
---|---|---|---|
bf-reactions-item-id | string | Yes | Unique ID for the Item where the reaction took place |
bf-reactions-group-name | string | No | A string representing the group name of the selected group of reactions defined in the reactions group feature. If not provided, the first available group in the reactions group feature will be used. If a not available group name is provided, it will throw an Error |
bf-reactions-item-type | string | No | A string representing the item type. This is useful in cases where different reactions are applied to different item types |
bf-reactions-show-count | boolean | no | If true , show Total reactions count |
bf-reactions-show-users-reactions | boolean | no | If true , show Users reactions when pressing on reactions count. Requires bf-reactions-show-count to be true . |
bf-reactions-on-update | string | no | An event handler function that will be called each time a reaction occurs. It will have an event parameter that is automatically passed, check the table below for more details. |
bf-reactions-on-error | string | no | An event handler function that will be called each time a reaction error occurs. It will have an event parameter that is automatically passed, check the table below for more details. |
bf-reactions-on-update event
Name | Type | Description |
---|---|---|
status | string | Could be one of the following: add , delete , update . add when performing a reaction, delete when we remove an active reaction, and update when we toggle an old reaction type with a new one |
reactionType | string | The reaction type of the reaction that was executed and fired the event |
itemId | string | Unique ID for the Item where the reaction took place |
userId | string | Unique ID for the logged-in user that made the reaction |
groupName | string | The Reaction group name that the reaction belongs to |
itemType | string | A string representing the item type. This is useful in cases where different reactions are applied to different item types |
reactionId | string | Unique ID for the logged-in user that made the reaction |
bf-reactions-on-error event
Name | Type | Description |
---|---|---|
itemType | string | A string representing the item type. This is useful in cases where different reactions are applied to different item types |
itemId | string | Unique ID for the Item where the reaction error took place |
errorCode | string | Error code, reflecting the error type and details |
message | string | Error description |
Examples
Using Javascript Methods
HTML
<div id="div-with-reactions"></div>
<script>
const reactions = new buildfire.components.reactions("#div-with-reactions",
{
itemId: "005589dfaeaab",
itemType: "post",
groupName: "Like", // This group name should be created in Reactions Feature
showCount: true,
showUsersReactions: true,
})
reactions.onUpdate = (event) => {
console.log(event);
}
reactions.onError = (error) => {
console.log(error.errorCode);
}
</script>
Using HTML Attributes
HTML
<div id="reactionsContainer">
<div
bf-reactions-item-id="005589dfaeaab1"
bf-reactions-item-type="post"
bf-reactions-on-update="onReaction" bf-reactions-on-error="onReactionError" bf-reactions-show-count="true" bf-reactions-show-users-reactions="true">
</div>
<div
bf-reactions-item-id="005589dfaeaab2"
bf-reactions-item-type="post"
bf-reactions-on-update="onReaction" bf-reactions-on-error="onReactionError" bf-reactions-show-count="true" bf-reactions-show-users-reactions="true">
</div>
<div
bf-reactions-item-id="005589dfaeaab3"
bf-reactions-item-type="post"
bf-reactions-on-update="onReaction" bf-reactions-on-error="onReactionError" bf-reactions-show-count="true" bf-reactions-show-users-reactions="true">
</div>
</div>
<script>
const onReaction = (event) => {
console.log(event);
}
const onReactionError = (error) => {
console.log(error.errorCode);
}
// Call the static method injectReactions() to search inside the selector for any element having the required reaction component attributes and render the corresponding reactions
buildfire.components.reactions.injectReactions("#reactionsContainer");
</script>
buildfire.components.control.reactionGroupPicker
Reaction Group Picker
The Reaction Group Picker component is a versatile tool that can be used to easily select one of the available reaction groups, defined in the Reactions feature.

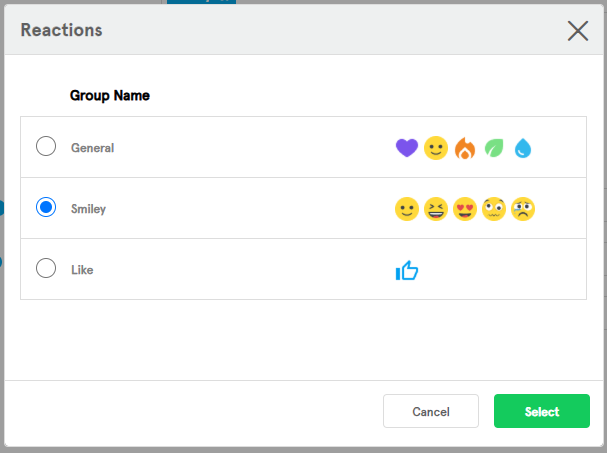
Requirements
Control
<head>
<!-- CSS -->
<link href="../../../../styles/helper.css" rel="stylesheet" />
<link href="../../../../styles/siteIcons.css" rel="stylesheet" />
<!-- JS -->
<script src="../../../../scripts/buildfire.min.js"></script>
<script src="../../../../scripts/buildfire/components/reactions/reactions.min.js"></script>
<script src="../../../../scripts/buildfire/components/control/reactions/reactionGroupsPicker.min.js"></script>
</head>
This component requires the Reactions feature to be installed in the app to function properly
Usage
The reactionGroupPicker
component takes care of fetching and displaying the available reactions groups and tracking selection group changes.
Reaction Group Picker
reactionGroupPicker()
const reactionsSelector = new buildfire.components.control.reactionGroupPicker(selector, options);
Class constructor method which initializes the reactionGroupPicker
component.
const reactionsSelector = new buildfire.components.control.reactionGroupPicker(selector<String>);
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
selector | string | yes | A string containing selector to match. This string must be a valid CSS selector string; if it isn't, a SyntaxError exception is thrown. | |
options | object | no | options object |
options
Name | Type | Required | Description | Default |
---|---|---|---|---|
placeholder | string | no | placeholder for the input field | Select Reaction Group |
groupName | string | no | Initial group name in the Reactions Groups picker |
Handlers
onUpdate()
An event handler function will be called each time a reaction group selection changes. It will have an event
parameter that is automatically passed.
reactionsSelector.onUpdate(event<Object>);
event
Name | Type | Description |
---|---|---|
name | string | Selected group name |
onError()
An event handler function will be called each time an error occurs. It will have an event
parameter that is automatically passed.
reactionsSelector.onError(event<Object>);
event
Name | Type | Description |
---|---|---|
errorCode | string | Error code, reflecting the error type and details |
message | string | Error description |
event.errorCode
Code | Description |
---|---|
1001 | Missing required data |
1002 | Invalid selector provided |
1003 | Provided group name does not exist |
5001 | Server failed to fetch data |
5002 | Server failed to update data |
5003 | Server failed to delete data |