buildfire.components.swipeableDrawer
Swipeable Drawer offers an interactive way to access extra content or features. Unlike the traditional Drawer that's fixed in size, the Swipeable Drawer comes to life with a user's swipe and offers resizable dimensions for more flexibility.
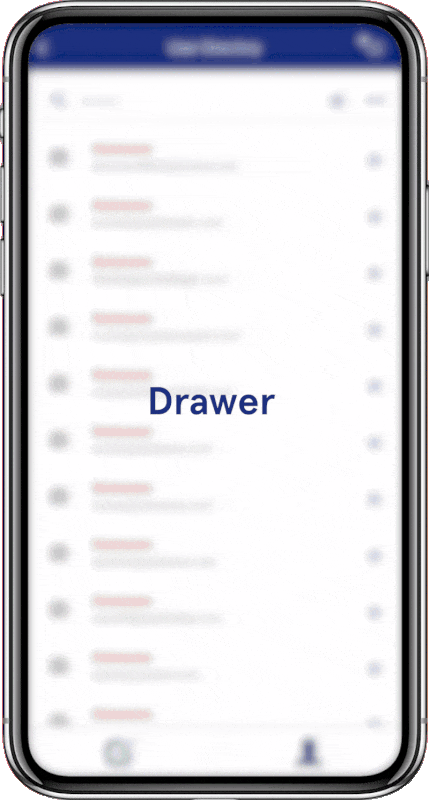
Requirements
After injecting the buildfire.min.js
inject the component css file swipeableDrawer.css
and js file swipeableDrawer.js
Widget
<head>
<!-- JS -->
<script src="../../../scripts/buildfire.min.js"></script>
<script src="../../../scripts/buildfire/components/swipeableDrawer/swipeableDrawer.js"></script>
<!-- CSS -->
<link rel="stylesheet" href="../../../styles/components/swipeableDrawer/swipeableDrawer.css" />
</head>
Methods
initialize()
buildfire.components.swipeableDrawer.initialize(options<Object>, callback<Function>)
Method which initializes the Swipeable Drawer component by passing options and provides a callback once the component has been rendered.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
options | object | false | Options object | |
callback | function | false | fires when the Swipeable Drawer is fully rendered and ready for use. It takes no parameters and returns void |
options
Name | Type | Required | Description | Default |
---|---|---|---|---|
startingStep | string | no | Determines the initial visibility level of the swipeableDrawer on the screen. Acceptable values are min for mostly hidden, mid for partially visible, and max for fully expanded. | min |
header | string or HTML | no | Header content of the component | |
content | string or HTML | no | Body content of the component | |
footer | string or HTML | no | Footer content of the component | |
transitionDuration | number | no | Transition duration expressed in milliseconds | 300 |
mode | string | no | Used for the behaviour of the component when a user interacts. free, steps | free |
backdropEnabled | boolean | no | Adds a backdrop to the component that when clicked will hide the drawer | false |
backdropShadow | string | no | A hex or rgba color string of the backdrop, only works when backdropEnabled is true | rgba(0, 0, 0, 0.50) |
Example
const drawerOptions = {
startingStep: "mid",
header: "<h4>Header Content</h4>",
content: "<p>Body Content</p>",
footer: "<div>Footer Content</div>",
mode: "steps",
transitionDuration: 500
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {});
show()
function buildfire.components.swipeableDrawer.show()
Displays the drawer component after injecting it into the DOM.
Example
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.hide();
setTimeout(() => buildfire.components.swipeableDrawer.show(), 1000);
});
hide()
function buildfire.components.swipeableDrawer.hide()
Hides the drawer component from the DOM
Example
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.hide();
});
setHeaderContent()
function buildfire.components.swipeableDrawer.setHeaderContent(content<String | HTML>)
Sets the HTML content to the drawer header element.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
content | string or HTML | yes | Use to pass desired string or HTML element |
Example
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.setHeaderContent("<h4>Header Content</h4>");
});
setBodyContent()
function buildfire.components.swipeableDrawer.setBodyContent(content<String | HTML>)
Sets the HTML content to the drawer body element.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
content | string or HTML | yes | Use to pass desired string or HTML element |
Example
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.setBodyContent("<p>Body Content</p>");
});
appendBodyContent()
function buildfire.components.swipeableDrawer.appendBodyContent(content<String | HTML>)
Appends the HTML content to the drawer body element.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
content | string or HTML | yes | Use to pass desired string or HTML element |
Example
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.appendBodyContent("<p>Additional Body Content</p>");
});
clearBodyContent()
function buildfire.components.swipeableDrawer.clearBodyContent()
Clears the HTML content of the drawer body element.
Example
const drawerOptions = {
startingStep: "mid",
content: "<p>Body Content</p>",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.clearBodyContent();
});
setFooterContent()
function buildfire.components.swipeableDrawer.setFooterContent(content<String | HTML>)
Sets the HTML content to the drawer header element.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
content | string or HTML | yes | Use to pass desired string or HTML element |
Example
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.setFooterContent("<div>Footer Content</div>");
});
setStep()
function buildfire.components.swipeableDrawer.setStep(step<String>)
Sets the current step of the drawer component
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
step | number | yes | min, mid, max |
This method will only work if the component mode is set to steps
.
Example
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.setStep("max");
});
Handlers
onStepChange()
function onStepChange(event<Object>)
Triggered when a user interacts with the component and changes its position
This handler will only trigger if the component mode is set to steps
.
Arguments
Name | Type | Required | Description | Default |
---|---|---|---|---|
event | object | yes | Contains search value and current sort option. |
event
Name | Type | Required | Description | Default |
---|---|---|---|---|
step | string | yes | Contains the current step of the component. |
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.onStepChange = (step) => console.log(step);
});
onHide()
function onHide()
Triggered when the drawer gets hidden
const drawerOptions = {
startingStep: "mid",
mode: "steps"
}
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.onHide = () => console.log('drawer is hidden');
});
Example
const content = `<div id="contentList">
<div class="drawer-item" style="border-bottom: 1px solid;" onclick="onItemClick()">
<img width="100" src="https://dummyimage.com/100x100/" >
<span>American cuisine</span>
</div>
<div class="drawer-item" style="border-bottom: 1px solid;" onclick="onItemClick()">
<img width="100" src="https://dummyimage.com/100x100/" >
<span>Cucina Urbana</span>
</div>
<div class="drawer-item" style="border-bottom: 1px solid;" onclick="onItemClick()">
<img width="100" src="https://dummyimage.com/100x100/" >
<span>Juniper & Ivy</span>
</div>
<div class="drawer-item" style="border-bottom: 1px solid;" onclick="onItemClick()">
<img width="100" src="https://dummyimage.com/100x100/" >
<span>The Fish Market</span>
</div>
</div>`;
window.onItemClick = (e) => {
console.log(`${e.target} clicked`);
};
const drawerOptions = {
startingStep: "max",
header: "<h4>Header Content</h4>",
content,
footer: "<div>Footer Content</div>",
mode: "steps",
transitionDuration: 500,
};
buildfire.components.swipeableDrawer.initialize(drawerOptions, () => {
buildfire.components.swipeableDrawer.show();
buildfire.components.swipeableDrawer.onStepChange = (step) => {
buildfire.components.swipeableDrawer.setHeaderContent(
`Current Step: ${step}`,
);
};
});